Use the following command:
apachectl start
Sunday, August 10, 2014
Why main method is static
Why we generally keep main() method in Java as static?
Because an object is not required to call the static method. Also till java7 static blocks if defined before main were executed standalone before the main method.
let's take an example:
if you look at the code below, you will see that Student.change(); calls the change method defined in the constructor without any dependency on objects like s1, s2, s3 and that's the beauty of static keyword in context of methods:
btw static variables are initialized only once and retains its values, a good example is as below, initially static variable count was = 0, when object c1 calls it, it increments to 1 and keep its value, if not defined static it would have lost its incremented value and when c2 object would have called the Counter constructor it would have received 1 again but because it is static and retains its value therefore on the next call of c2 it increments to 2 from 1 and 3 from 2 when c3 object calls the constructor.
Because an object is not required to call the static method. Also till java7 static blocks if defined before main were executed standalone before the main method.
let's take an example:
if you look at the code below, you will see that Student.change(); calls the change method defined in the constructor without any dependency on objects like s1, s2, s3 and that's the beauty of static keyword in context of methods:
- //Program of changing the common property of all objects(static field).
- class Student{
- int rollno;
- String name;
- static String college = "ITS";
- static void change(){
- college = "BBDIT";
- }
- Student(int r, String n){
- rollno = r;
- name = n;
- }
- void display (){System.out.println(rollno+" "+name+" "+college);}
- public static void main(String args[]){
- Student.change();
- Student s1 = new Student (111,"Karan");
- Student s2 = new Student (222,"Aryan");
- Student s3 = new Student (333,"Sonoo");
- s1.display();
- s2.display();
- s3.display();
- }
- }
Output:111 Karan BBDIT 222 Aryan BBDIT 333 Sonoo BBDIT
btw static variables are initialized only once and retains its values, a good example is as below, initially static variable count was = 0, when object c1 calls it, it increments to 1 and keep its value, if not defined static it would have lost its incremented value and when c2 object would have called the Counter constructor it would have received 1 again but because it is static and retains its value therefore on the next call of c2 it increments to 2 from 1 and 3 from 2 when c3 object calls the constructor.
- class Counter{
- static int count=0;//will get memory only once and retain its value
- Counter(){
- count++;
- System.out.println(count);
- }
- public static void main(String args[]){
- Counter c1=new Counter();
- Counter c2=new Counter();
- Counter c3=new Counter();
- }}
Output:1 2 3
static keyword in Java
static keyword is being used in the JAVA world extensively, reason for this use us as follows:
1. static keyword has to do with the memory management.
2. The static variable gets memory only once in class area at the time of class loading.
a very classic example of point2 is as below:
if you write test cases in JUnit, you may come across @BeforeClass, @AfterClass, @Before, @After annotations. As you must be aware that @BeforeClass and @AfterClass are executed only once, @BeforeClass at the time of class loading, @AfterClass after all the test cases are executed, therefore it is safe to use static keyword with any method that has to be executed for @BeforeClass or @AfterClass.
You may also be aware that @Before methods and @After methods are executed for each @Test method i.e. in JUnit test cases are executed between @Before and @After, if you try to use the static keyword with any method associated with @Before or @After it will result into a compiler error. Try this code in eclipse where JUnit is setup properly and to see the compiler error put static keyword for methods defined inside @Test annotation or @Before annotation or @After annotation:
if you remember, we always use static keyword while defining main in Java, reason is that main need to be executed only once:
public static void main(String args[])
A very nice tutorials to completely understand about "static" and refer again and again is - http://www.javatpoint.com/static-keyword-in-java
1. static keyword has to do with the memory management.
2. The static variable gets memory only once in class area at the time of class loading.
a very classic example of point2 is as below:
if you write test cases in JUnit, you may come across @BeforeClass, @AfterClass, @Before, @After annotations. As you must be aware that @BeforeClass and @AfterClass are executed only once, @BeforeClass at the time of class loading, @AfterClass after all the test cases are executed, therefore it is safe to use static keyword with any method that has to be executed for @BeforeClass or @AfterClass.
You may also be aware that @Before methods and @After methods are executed for each @Test method i.e. in JUnit test cases are executed between @Before and @After, if you try to use the static keyword with any method associated with @Before or @After it will result into a compiler error. Try this code in eclipse where JUnit is setup properly and to see the compiler error put static keyword for methods defined inside @Test annotation or @Before annotation or @After annotation:
import org.junit.BeforeClass;
import org.junit.Before;
import org.junit.After;
import org.junit.AfterClass;
import org.junit.Test;
import org.junit.Ignore;
public class JunitExecution {
@BeforeClass
public static void BeforeClass()
{
System.out.println("Entering
BeforeClass");
}
@AfterClass
public static void AfterClass()
{
System.out.println("Entering
AfterClass");
}
@Before
public void Before()
{
System.out.println("Entering
Before");
}
@After
public void After()
{
System.out.println("Entering
After");
}
@Test
public void test1()
{
System.out.println("Inside
Test1");
}
@Test
public void test2()
{
System.out.println("Inside
Test2");
}
@Ignore
public void ignore()
{
System.out.println("Inside
ignore");
}
}
if you remember, we always use static keyword while defining main in Java, reason is that main need to be executed only once:
public static void main(String args[])
A very nice tutorials to completely understand about "static" and refer again and again is - http://www.javatpoint.com/static-keyword-in-java
Resources - Selenium and JUNIT
I'm doing some extensive reading/research about this subject so I'll share the good articles/slidshows/books/answers I found:
- http://www.qaautomation.net/?cat=7 : I used this website & I thought it was pretty good (for Selenium anyway).
- http://www.vogella.com/articles/JUnit/article.html : just had a look & seems interesting
- wakaleo: junit-kung-fu-getting-more-out-of-your-unit-tests : a great overview on some key JUnit features
- testing-a-web-application-with-selenium-2/ : Selenium + JUnit
- SO question: how-to-use-junit-and-hamcrest-together (TBC) Be careful when using JUnit & Hamcrest together: if Hamcrest has a version greater or equal to 1.2, then you should use the junit-dep.jar. This jar has no hamcrest classes and therefore you avoid classloading problems.
- Moreover regarding Hamcrest, it states on its google code page that "Hamcrest it is not a testing library: it just happens that matchers are very useful for testing". Hence you may want to look into FEST "whose mission is to simplify software testing".
- This book came out recently (Published in 2012 vs lots of out-of-date books) & it looks very interesting: Practical Unit Testing with TestNG and Mockito by Tomek Kaczanowski
Thursday, August 7, 2014
Processing the request
doGet(request, response)
response.setContentType("text/html")
request.getParameters()
doPost(request, response)
response.setContentType("text/html")
request.getParameters()
doPost(request, response)
Wednesday, August 6, 2014
Why Tomcat
I wanted to execute a code written in Java rather than PHP or JS on the server side. I came to know that tomcat is a server that understand how to execute Java code on the server side and does it with the help of servlets. That's the way I landed into this world of Java servlets and tomcat otherwise PHP/JS is my favorite language to be used at server-side. To be frank, I have never used other than these two language on the server-side so far. If you are also going to start on java servlets like me, i am using the tutorial here and its nice - - http://www.tutorialspoint.com/servlets/servlets-environment-setup.htm
Starting with tomcat setup
Let's start with the tomcat setup where I did following:
DOWNLOAD:
Downloaded tomcat from http://tomcat.apache.org/.
I downloaded the version 8 that was the latest version
INSTALL:
Get the zip directory and unzip it into some folder like C:\folder1\folder2\tomcat8
After unzip, move to the bin directory and run the startup.bat and access http://localhost:8080 to make sure that you see that tomcat welcome page. It means things are moving in the right direction.
SYSTEM VARIABLES
Set the system variable - CATALINA_HOME to C:\folder1\folder2\tomcat8
Go to cmd and type echo %CATALINA_HOME% to see it emitting the correct folder that you have specified, it will confirm that the env variable has set correctly.
Now go to the system variable PATH and add - %CATALINA_HOME%\bin
Go back and do windows+R and type startup.bat, it should start the tomcat server.
Access the page http://localhost:8080 to make sure that you see that tomcat welcome page. It means things are moving in the right direction.
HOSTING
Go to your %CATALINA_HOME%\webapps\ROOT folder and create test.html page
Access this page by http://localhost:8080.test.html to make sure that you see your test page. It means things are moving in the right direction.
Server Status
On the welcome page, clicking on server status will ask you for the credentials. There are no default credentials, go to %CATALINA_HOME%\conf\tomcat-users.xml and edit it in a editor. Add the following line in the last:
<role rolename="manager-gui"/>
<user username="tomcat" password="tomcat" roles="manager-gui"/>
save it and restart the tomcat. Now using the tomcat/tomcat credentials you should be able to access the page. Roles and their meanings are as below:
Configure Tomcat Ports
The most common hiccup is when another web server (or any process for that matter) has laid claim to port 8080. This is the default HTTP port that Tomcat attempts to bind to at startup. To change this, open the file:
$CATALINA_HOME/conf/server.xml
and search for '8080'. Change it to a port that isn't in use, and is greater than 1024, as ports less than or equal to 1024 require superuser access to bind under UNIX.
Restart Tomcat and you're in business. Be sure that you replace the "8080" in the URL you're using to access Tomcat. For example, if you change the port to 1977, you would request the URL http://localhost:1977/ in your browser.
DOWNLOAD:
Downloaded tomcat from http://tomcat.apache.org/.
I downloaded the version 8 that was the latest version
INSTALL:
Get the zip directory and unzip it into some folder like C:\folder1\folder2\tomcat8
After unzip, move to the bin directory and run the startup.bat and access http://localhost:8080 to make sure that you see that tomcat welcome page. It means things are moving in the right direction.
SYSTEM VARIABLES
Set the system variable - CATALINA_HOME to C:\folder1\folder2\tomcat8
Go to cmd and type echo %CATALINA_HOME% to see it emitting the correct folder that you have specified, it will confirm that the env variable has set correctly.
Now go to the system variable PATH and add - %CATALINA_HOME%\bin
Go back and do windows+R and type startup.bat, it should start the tomcat server.
Access the page http://localhost:8080 to make sure that you see that tomcat welcome page. It means things are moving in the right direction.
HOSTING
Go to your %CATALINA_HOME%\webapps\ROOT folder and create test.html page
Access this page by http://localhost:8080.test.html to make sure that you see your test page. It means things are moving in the right direction.
Server Status
On the welcome page, clicking on server status will ask you for the credentials. There are no default credentials, go to %CATALINA_HOME%\conf\tomcat-users.xml and edit it in a editor. Add the following line in the last:
<role rolename="manager-gui"/>
<user username="tomcat" password="tomcat" roles="manager-gui"/>
save it and restart the tomcat. Now using the tomcat/tomcat credentials you should be able to access the page. Roles and their meanings are as below:
- manager-gui — Access to the HTML interface.
- manager-status — Access to the "Server Status" page only.
- manager-script — Access to the tools-friendly plain text interface that is described in this document, and to the "Server Status" page.
- manager-jmx — Access to JMX proxy interface and to the "Server Status" page.
Configure Tomcat Ports
The most common hiccup is when another web server (or any process for that matter) has laid claim to port 8080. This is the default HTTP port that Tomcat attempts to bind to at startup. To change this, open the file:
$CATALINA_HOME/conf/server.xml
and search for '8080'. Change it to a port that isn't in use, and is greater than 1024, as ports less than or equal to 1024 require superuser access to bind under UNIX.
Restart Tomcat and you're in business. Be sure that you replace the "8080" in the URL you're using to access Tomcat. For example, if you change the port to 1977, you would request the URL http://localhost:1977/ in your browser.
Introduction
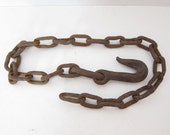
Subscribe to:
Posts (Atom)